Download package
wget https://www.tana.it/sw/zdkimfilter/zdkimfilter-3.8.tar.gz
Install necessary packages
sudo apt install pkg-config libbsd-dev libssl-dev zlib1g-dev libunistring-dev libidn2-dev libopendbx1-dev
Compile and install
tar xfz zdkimfilter-3.8.tar.gz cd zdkimfilter-3.8 ./configure make sudo make install
Configure
Get superpowers :
sudo -i
Generate keys
cd /etc/courier/filters mkdir keys chown courier:courier keys cd keys /usr/local/bin/zdkimgenkey -s mydomain -d thisdomain.org
Here, the selector mydomain is just a name that I chose different from the real domain thisdomain.org for the purpose of this tutorial. You would normally use something like thisdomain.
chown courier:courier *
Setup the DNS
You have to put the public key that you just generated (the mydomain.txt file) into your DNS. It is provided in a bind-compatible format. Since I use djbdns, I have to transform the entry. I use the site https://andersbrownworth.com/projects/sysadmin/djbdnsRecordBuilder/#domainKeys to do the dirty work :
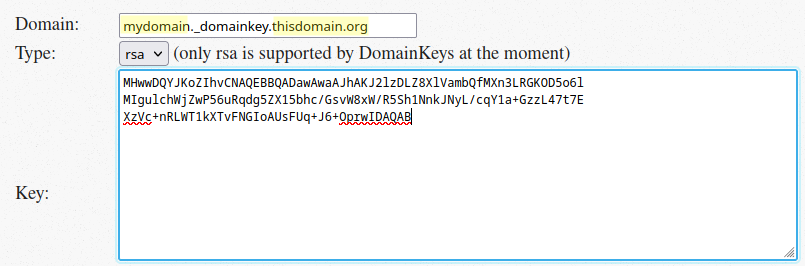
Just enter what follows p= in the TXT.
Configure
cd .. mv zdkimfilter.conf.dist zdkimfilter.conf
Add a default domain at the end of the config file. I use thisdomain.org but you can use any domain. It is used when zdkimfilter cannot guess the real domain, that is, when the sender is something like paul (without @somedomain.tld).
It is recommended to also add the following lines :
no_signlen = Y header_canon_relaxed = Y body_canon_relaxed = Y
Create the links and start the filter
cd keys ln -s mydomain.private thisdomain.org filterctl start zdkimfilter